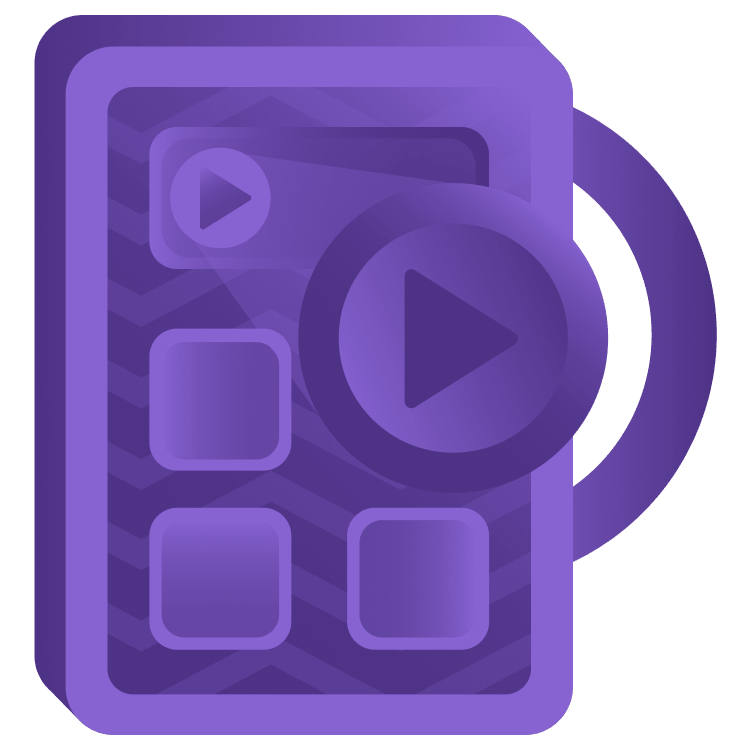
Creating Shortcuts with App Intents
Learn how to create iOS shortcuts using Swift in this App Intents tutorial. By Mark Struzinski.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Creating Shortcuts with App Intents
25 mins
Adding shortcuts support can be a great way to differentiate your app and offer excellent integration into the iOS ecosystem. Shortcuts allow your users to interact with your app without launching and navigating into specific screens to perform tasks. Shortcuts can use voice, text or Spotlight to accomplish quick tasks without much mental overhead. Your users can also build larger workflows with your shortcuts to accomplish many tasks simultaneously.
In the past, adding Shortcuts and exposing them to the system could be cumbersome and time-consuming. After your shortcut was set up and available, you had to figure out the best way to inform the user that it existed and show how to use it. In iOS 16, Apple revamped the process of adding and exposing your app’s Shortcuts to iOS. The old process of adding Shortcuts included finicky visual editors and mapping code files to Intent Definition files.
The new App Intents framework lets you create shortcuts from the same language you use daily – Swift! Everything is statically typed and ingested into iOS upon installation. Your shortcuts are immediately available to users via Siri, the Shortcuts app and Spotlight.
It’s time to dive into this new simple way to add Shortcuts to the system!
Getting Started
Download the starter project by clicking the Download Materials button at the top or bottom of the tutorial.
The BreakLogger app allows you to record break times throughout the day. Open the starter app project. Build and run BreakLogger. You’ll see an empty break list:
Tap the Add button at the bottom of the screen, and you’ll be prompted to record a break:
Select a break to add. Next, you’ll see a list of the breaks you’ve added — including the one you just recorded:
Right now, the only way to log a break is from within the app. But iOS has several other ways to interact with apps throughout its ecosystem. Users may want to log a break as part of a larger workflow, combining several actions into one shortcut. They may also want to tell Siri to log a break without opening the app while on the go. These use cases are possible if you integrate the App Intents framework into your app.
iOS 16 introduced the App Intents framework. Shortcut definitions are now built entirely in Swift, with no code generation or additional steps needed to make them available across iOS. You no longer need to use Intent Definition files or the visual editors. Get started building your own with your first App Intent.
Defining Your First App Intent
Inside the starter project, right-click the Source group and select New Group. Name the group Intents. Inside the new group, right-click again and select New File… . Select the Swift File template and choose Next. Name the file LogBreakIntent.swift.
Create a LogBreakIntent
struct that conforms to AppIntent
:
import AppIntents
import SwiftUI
// 1
struct LogBreakIntent: AppIntent {
// 2
static let title: LocalizedStringResource = "Log a Break"
// 3
func perform() async throws -> some IntentResult & ProvidesDialog {
// 4
let loggerManager = LoggerManager()
loggerManager.logBreak(for: .quarterHour)
// 5
return .result(dialog: "Logged a 15 minute break")
}
}
Here’s what’s happening in the code above:
- Creates a
struct
to represent your shortcut. Adds conformance to theAppIntent
protocol. - Adds a
title
property. This is of typeLocalizedStringResource
, which makes the string available out of process for localization lookup. Remember this code also runs when your app isn’t in memory. - Adds a
perform()
function to finalize conformance to theAppIntent
protocol. Indicates it returns anIntentResult
and that it provides a dialog. - Uses the
LoggerManager
convenience type to log a 15-minute break. This action also saves the break to the Core Data store. - Returns the
IntentResult
as a dialog.
With the current setup, a user can create a shortcut and the intents from BreakLogger will appear as available actions. These actions run by themselves or as part of a larger shortcut composed of many actions. As soon as a user installs BreakLogger your intents are available as shortcut actions.
Build and run to ensure the updated code installs to the simulator. Next, background the app with the keyboard shortcut Command + Shift + H
. You’ll see the Shortcuts app on the same screen. Open Shortcuts.
Tap the + button on the top right. The New Shortcut view loads.
On the New Shortcut view, tap Add Action. In the segmented control at the top, select Apps.
Select BreakLogger from the list, then tap Log a Break.
Tap Done. The view dismisses and takes you back to the Shortcuts tab in the app. You’ll see your new shortcut on the screen now. You’ve created a shortcut with your first app intent!
Tap the Log a Break shortcut to run it. You’ll see a dialog drop down after a second or two with the content you set up in the perform()
function.
Tap Done, close Shortcuts and open BreakLogger again. You’ll see the break you just logged added to the list.
Well done! You added Shortcuts integration into your app with a few Swift files in a few steps. Next, you’ll add an app shortcut to BreakLogger.
Adding an App Shortcut
Back in Xcode, add a new Swift file to the Intents group you created earlier. Name the file BreakLoggerShortcuts.swift.
Add the following:
import AppIntents
// 1
struct BreakLoggerShortcuts: AppShortcutsProvider {
// 2
static var appShortcuts: [AppShortcut] {
// 3
AppShortcut(
// 4
intent: LogBreakIntent(),
// 5
phrases: [
"Log a break",
"Log a \(.applicationName) break"
]
)
}
}
Here’s what this code does:
- Creates a struct that conforms to
AppShortcutsProvider
. This lets iOS know to index the shortcuts here. - Adds a static property that returns an array of
AppShortcut
instances. For this example, you’ll only be providing one. - Creates an
AppShortcut
. - Uses
LogBreakIntent
as the intent behind the shortcut. - Provides a list of phrases that can trigger this shortcut. Note the use of the special token
.applicationName
, which resolves to the localized name of your app.
Now, build and run.
Background the app. You’ll be able to start Siri on the simulator and say, “Use BreakLogger to a break” and have the shortcut run immediately.
You’ll see a prompt asking you to enable BreakLogger shortcuts with Siri:
Then, you’ll see a confirmation dialog:
Finally, open BreakLogger again. You’ll see your second logged break from the shortcut you just ran.
Next, you’ll create a custom confirmation view to show whenever the intent has run.