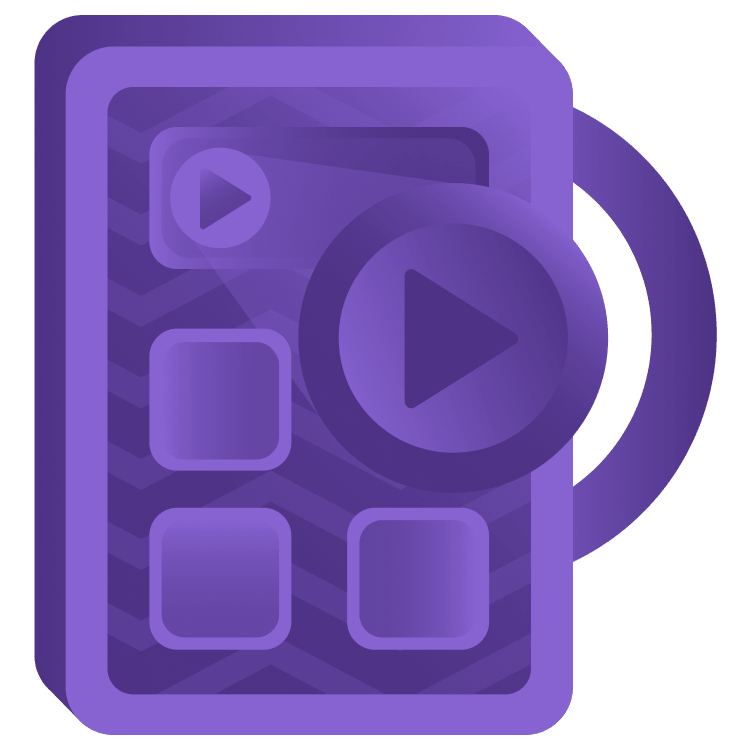
Creating Shortcuts with App Intents
Learn how to create iOS shortcuts using Swift in this App Intents tutorial. By Mark Struzinski.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Creating Shortcuts with App Intents
25 mins
Using Parameterized Phrases
Parameterized phrases allow your users to speak natural language phrases to Siri and immediately perform actions with your shortcuts. Adding support for these phrases lets the user skip a disambiguation step if they know exactly what they want. Siri doesn’t support dynamic phrases with freeform parameters. But if your app has a finite list of potential parameters, you can generate phrases based on these values and provide them as quick actions to your users.
Since BreakLogger has a small list of parameters, you’ll implement some phrases now.
First, you need to tell the EntityQuery
what phrases are possible for suggestion. Open LogBreakQuery.swift and add a suggestedEntities()
function at the end of the struct:
func suggestedEntities() async throws -> [BreakIncrementEntity] {
LoggerManager.allBreakIncrements()
}
Although this function isn’t required for conformance to EntityQuery
, if you don’t include it, parameterized phrases won’t show up.
Next, let App Intents know about your parameterized phrases by calling updateAppShortcutParameters()
on BreakLoggerShortcuts
. iOS generates this function for you. You don’t have to implement it on your own. Since LoggerManager
initializes on every app run, it makes sense to put this call in the initialization of this struct. Open LoggerManager.swift and override init()
to add this maintenance code:
init() {
BreakLoggerShortcuts.updateAppShortcutParameters()
}
Finally, you need to define the list of parameterized phrases. You’ll add one additional phrase to BreakLoggerShortcuts
. This will trigger App Intents to generate a separate phrase for each value of your parameter list. Open BreakLoggerShortcuts.swift and update the body of the computed appShortcuts
var to the following:
AppShortcut(
intent: LogBreakIntent(),
phrases: [
"Log a break",
"Use \(.applicationName) to log a break",
// 1
"Start a \(\.$breakIncrement) break with \(.applicationName)"
]
)
The code does the following:
- You’re adding a parameterized phrase here. This will use each potential value of
BreakIncrementEntity
to generate unique phrases for Siri. Each phrase uses the.applicationName
token and a keypath tobreakIncrementEntity
to create a phrase. The entity will use itsdisplayRepresentation
property as its value for the phrase.
That’s all it takes to incorporate parameterized phrases into the app. Build and run.
After the app launches, send it to the background and open Shortcuts. You’ll see a shortcut for each variation of the BreakEntity
parameter:
Next, wrap up with some methods to help users discover your shortcuts more easily.
Adding Usage Tips
iOS 16 added SiriTipView. This view replaced the Add to Siri button, which is no longer needed. iOS now adds shortcuts to the system on app launch using the App Intents framework. The tip view is a good way to educate users on shortcut availability in a contextual way.
For example, when someone navigates to the Add Break screen of BreakLogger, it would be good to give them a hint that they can also add a break with a shortcut. This is exactly what SiriTipView
does.
Open AddBreakView.swift.
Add an import for App Intents at the top of the file:
import AppIntents
Just below the view declaration, add a state variable to keep track of the tip view’s visibility:
@State private var tipIsShown = true
Then, under the first Text
view, add a SiriTipView
:
SiriTipView(
intent: LogBreakIntent(),
isVisible: $tipIsShown
)
.padding(10.0)
SwiftUI will manage the tipIsShown
value if the user closes the tip view during an app session. If you want the tip view to remain hidden in a more persistent fashion, write some state to UserDefaults and keep track of it there.
Add a Spacer()
at the bottom of the VStack
to push the content to the top of the view.
Build and run. Navigate to AddBreakView
. You’ll see the SiriTipView
at the top of the screen with a close button on the trailing edge:
Finally, another way to educate users about your shortcuts is the new ShortcutsLink view. This view will link out to your app’s page in the Shortcuts app and show your users all available shortcuts.
Still in AddBreakView.swift, add a ShortcutsLink
under the Spacer() inside the VStack
:
ShortcutsLink()
Build and run. You’ll see the ShortCutsLink
at the bottom of the view:
Where to Go From Here
Download the completed project files by clicking the Download Materials button at the top or bottom of the tutorial.
The new App Intents framework in iOS 16 makes supporting Shortcuts easier and more straightforward. The code-based approach for all Shortcuts, Spotlight and Siri integrations keeps you in Swift. This makes your implementation quicker and easier to reason about. There are other areas of App Intents to explore that aren’t covered here.
Research how to add custom views for disambiguation and run confirmation for shortcuts as well. iOS 17 adds some new capabilities for App Intents, too. Widgets are now included as a beneficiary of the new intent setup. Check out Explore Enhancements to App Intents for more information.
We hope you enjoyed this tutorial. If you have any questions or comments, please join the forum discussion below!