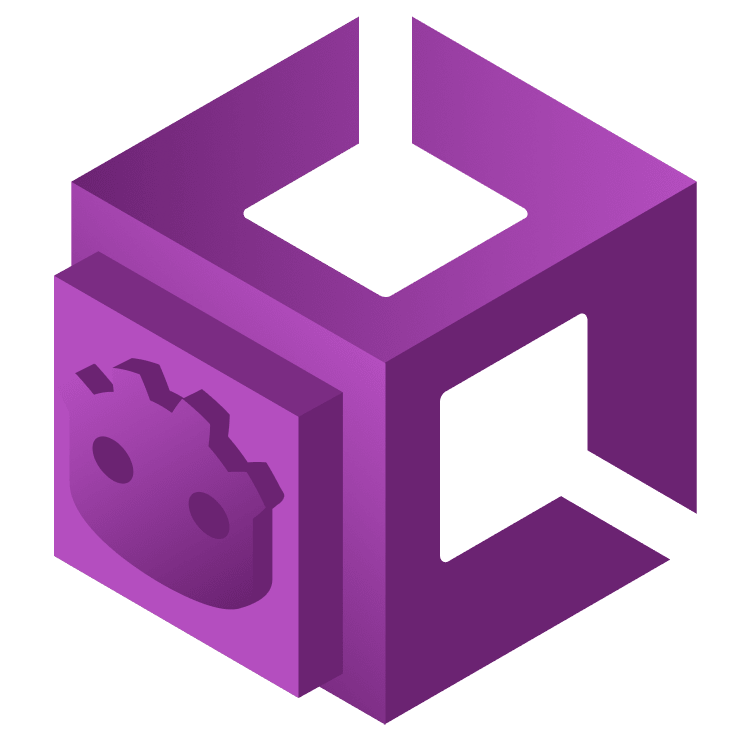
Getting Started with Godot for Unity Developers
Are you a Unity game developer curious about Godot? While Unity still dominates the market, Godot is rapidly gaining ground. Exploring multiple game engines can provide invaluable insights into their strengths and weaknesses. Godot, known for its ease of use, open-source nature, and recent 3D enhancements in Godot 4, offers an attractive alternative. By Eric Van de Kerckhove.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Getting Started with Godot for Unity Developers
25 mins
Design Comparison
Unity uses an Entity Component System (ECS) to manage its objects, while Godot uses a node-based system that aligns more closely with conventional object-oriented programming (OOP). This contrast encourages you to approach game structure from a different perspective. It’s important to note that neither approach is inherently superior; it’s a matter of personal preference.
Nodes
The most basic building block in Unity is a GameObject, a container for a collection of components. In Godot, a node fulfills a similar role, but each node has a specific purpose.
To illustrate this with an example: imagine you have a 2D player object that needs to move around and has an amount of health. In Unity, you’d create a Player GameObject and attach some components:
- Sprite Renderer component to render a sprite
- RigidBody2D component to move the player around
- Capsule Collider 2D component to detect collisions and define the collision shape
- Custom Health component to manage the health
In Godot, you’d create a chain of nodes:
-
RigidBody2D node as the root node to move the player around and detect collisions. It has the following nodes as its children:
- Sprite2D node to render a sprite
- CollisionShape2D node to define the collision shape
- A regular Node with a custom Health script attached to manage the health
- Sprite2D node to render a sprite
- CollisionShape2D node to define the collision shape
- A regular Node with a custom Health script attached to manage the health
Another difference that’s harder to spot at a first glance is that most components in Unity use unique scripts to provide their functionality, whereas Godot’s nodes use inheritance. Take the Sprite2D node for example. Its inheritance chain is: Object ▸ Node ▸ CanvasItem ▸ Node2D ▸ Sprite2D.
This means a Sprite2D nodes inherits the properties of Node2D, which in turn inherits the properties of CanvasItem, and so on. This makes it easier to reuse common functionality across nodes. In Unity, it’s the Transform component that provides a position in the scene, whereas in Godot, it’s the Node2D that has a Position property.
The main concept to keep in mind is that Godot’s nodes are like components in Unity, but instead of needing a GameObject to attach to, they need another node as a parent.
Scenes
In Unity, a scene comprises a collection of GameObjects, whereas in Godot, scenes are a collection of nodes. At first glance, they may seem similar, but there are important distinctions to consider.
You can compare a Godot scene to a prefab you would create in Unity, as Godot scenes can be instantiated. Moreover, Godot scenes can be nested within other scenes and have the ability to inherit from other scenes. In contrast, in Unity, you’d typically create a GameObject and save it as a prefab for future use, whereas in Godot, you’d construct a node tree and save it as a scene for later.
Here’s a small code example of how instantiating a scene works in Godot:
var my_scene_instance = load("res://my_scene.tscn").instantiate()
add_child(my_scene_instance)
The scene gets loaded from the disk and then instantiated as a child of the current node. This is the same as instantiating a prefab in Unity:
public GameObject myPrefab;
void Start()
{
var myInstance = Instantiate(myPrefab, new Vector3(0, 0, 0), Quaternion.identity);
myInstance.transform.parent = transform;
}
Because scenes in Godot are files, you can even load them from the web! This opens up a lot of creative possibilities for game development.
In summary, scenes in Godot are files that contain a node tree. You can compare them to both scenes and prefabs in Unity.
Signals
There are a multiple ways of using events in Unity. These are useful when you want something to happen as a reaction to something else. For example, you might want to play a sound when a character’s health falls below a certain threshold. Here are some ways Unity allows you to set up events:
-
UnityEvent: This is used for buttons for example, where you can link the
OnClick()
event to a method. You can also create custom events. -
Built-in functions: To check for collisions with a trigger, you would add a
OnTriggerEnter(Collider)
method. - Delegates: In simple terms, a delegate is a method container. You can add methods to the delegate and when the delegate is called, all the methods are called. This can be used to create custom event handlers, for when a character loses health for example.
These are all examples of the Observer pattern, where a subscription mechanism notifies its subscribers when an event occurs.
In Godot, events are handled via a signal system. Every node in Godot has one or more signals it emits in response to events. A button for example can emit a signal when pressed or when the cursor hovers over it.
The beauty of this system is that it’s consistent across all nodes. A RigidBody2D node has a body_entered
signal, a Sprite2D node has a frame_changed
signal, and so on. There’s no need to remember what kind of event system you need per use case, unlike in Unity.
Subscribing to signals as easy as it gets. You can either link a signal to a function via the user interface or with one line of code:
button.pressed.connect(make_a_sound)
Creating custom signals is also trivial:
signal my_signal
func something_cool_happened():
my_signal.emit()
You can also pass values with the signals:
signal health_changed(old_health, current_health)
func hit_by_enemy(damage):
var old_health = health
health -= amount
health_changed.emit(old_health, health)
In my opinion, Godot’s signal system is vastly superior to Unity’s multiple ways of handling events. It’s clean, easy to understand and works as expected. If you want to know more about signals, you can read more in Godot’s Signal documentation.
Animations
Unity uses the Mecanim animation system and the Animator component to animate anything from characters to UI element and variables.
On the Godot side, you can use an AnimationPlayer node to animate anything, just like in Unity.
Both animation systems allow you to animate properties over time and call functions at specific points in time. If you’ve ever used the Animation window in Unity, you’ll feel right at home with Godot’s AnimationPlayer and the Animation dock. For more information, check out the Introduction to Godot animation features page.
When it comes to 3D humanoid animations, Unity uses the Avatar system to retarget animations to different characters. This means you can create a single animation for multiple characters with different body shapes. You can see an example of how this works in one of my earlier tutorials. Godot has a Skeleton3D node that offers the same functionality, including automatically mapping bones to body parts based on their name. To learn more, take a look at Godot’s Retargeting 3D Skeletons page.